5.7. Help and doc strings¶
There are a number of basic Python and IPython help facilities, which can help you learn more about Python objects and functions.
5.7.1. Basic Python help¶
Start with the help
function:
1 >>> help(list)
2Help on class list in module __builtin__:
3
4class list(object)
5 | list() -> new empty list
6 | list(iterable) -> new list initialized from iterable's items
7 |
8 | Methods defined here:
9 |
10 | __add__(...)
11 | x.__add__(y) <==> x+y
12 |
13 | __contains__(...)
14 | x.__contains__(y) <==> y in x
15 |
16 | __delitem__(...)
17 | x.__delitem__(y) <==> del x[y]
18 |
19 | __delslice__(...)
20 | x.__delslice__(i, j) <==> del x[i:j]
21 |
22 | Use of negative indices is not supported.
23 |
24 | __eq__(...)
25| x.__eq__(y) <==> x==y
26 |
27 | __ge__(...)
28 | x.__ge__(y) <==> x>=y
29 |
30 | __getattribute__(...)
31 | x.__getattribute__('name') <==> x.name
32 |
33 | __getitem__(...)
34 | x.__getitem__(y) <==> x[y]
35 |
36 | __getslice__(...)
37 | x.__getslice__(i, j) <==> x[i:j]
38 |
39 | Use of negative indices is not supported.
40 |
41 | __gt__(...)
42 | x.__gt__(y) <==> x>y
43 |
44 | __iadd__(...)
45 | x.__iadd__(y) <==> x+=y
46 |
47 | __imul__(...)
48 ...
This particular help
string
has a lot more information, more omitted here.
Most Python builtin functions have documentation
strings stored on an attribute __doc__
,
so you can read doc strings like this:
1>>> print len.__doc__
2
3len(object) -> integer
4
5Return the number of items of a sequence or mapping.
The same is true of modules. Once a module is loaded,
its documentation string can be printed as above.
For example, documentation for the regular expression module,
re
, which
will be extensively covered in the next chaoter, can be
accessed as follows:
1>>> import re
2>>> print re.__doc__
3Support for regular expressions (RE).
4
5This module provides regular expression matching operations similar to
6those found in Perl. It supports both 8-bit and Unicode strings; both
7the pattern and the strings being processed can contain null bytes and
8characters outside the US ASCII range.
9
10Regular expressions can contain both special and ordinary characters.
11Most ordinary characters, like "A", "a", or "0", are the simplest
12...
For any module or any class instance, you can learn the
set of functions defined in the module or the set of attributes
of the class, by using the dir
(directory) function. For
example, let’s take take an instance of the list type:
1>>> L = ['a']
2>>> dir(L)
3['__add__', '__class__', '__contains__', '__delattr__', '__delitem__',
4 '__delslice__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__',
5 '__getitem__', '__getslice__', '__gt__', '__hash__', '__iadd__', '__imul__',
6 '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__',
7 '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__',
8 '__setattr__', '__setitem__', '__setslice__', '__sizeof__', '__str__',
9 '__subclasshook__', 'append', 'count', 'extend', 'index', 'insert', 'pop',
10 'remove', 'reverse', 'sort']
Thus we get at the same set of methods listed by the help function, but without the documentation, sometimes an easier way to get at the information when you know what you’re looking for.
Python’s online documentation is extensive, and is usually the first thing
listed in the Google search results for some Python function or module
accompanied by the word Python
.
5.7.2. Basic IPython help¶
IPython
provides an extended help, using the question mark
operator. See how to use
the question mark operator, as well other IPython help
facilities, see the IPython docs.
In addition, when you are typing a function and its arguments in the IPython console or a notebook, if you pause after you type the left parentheses, a small help window appears with function documentation, if available.
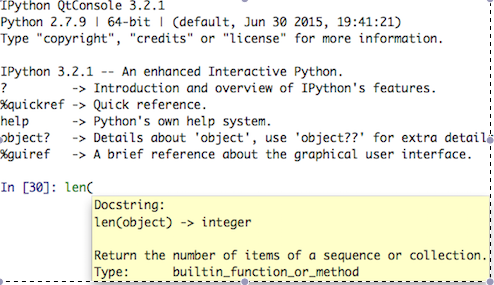